Understand CKAuth. key principles.
Your client application requests user authentication credentials from the CrossKnowledge Authorization Server, then request an access token to the CrossKnowledge Authentication Server, extracts a token from the response, and sends the token to the CrossKnowledge Learner API that you want to access.
Basic steps.
All applications follow a basic pattern when accessing a CrossKnowledge Learner API using CKAuth. At a high level, you follow four steps:1.Obtain an authentication token from the CrossKnowledge Authorization Server.
Before your client application can access user data using CrossKnowledge Learner API, it must obtain an authentication token that grants access to the API. There are several ways to make this requests.
For example, your application can ask to the user to provide its 6 digits authorization code that will be used to get an authentication token on it's behalf. Or, your application can request for a 6 digits code by using the user identifier.
If the CrossKnowledge Authorization Server grants the permission, it will sends back an authentication token to your application that will be used to get an access token to the API.
2.Obtain an access token from the CrossKnowledge Authentication Server.
After your application obtains an authentication token, it sends the token to a CrossKnowledge Authentication Server using an HTTP POST
request. The authentication server will then respond with an access token that will be used by your application to call CrossKnowledge API web-services.
The access token is only valid for the current authenticated user on your application. This means it can't be used to get private data from another user.
3.Get user data using CrossKnowledge Learner API.
Now that your application owns a valid access token, it can get user data using the CrossKnowledge Learner API web-services. Your application can call any of the web-services available using that access token.
Authentication and Authorization workflow
1. Application authorization based on user authorization code.
In this scenario, your application must use the 6 digits code provided by the user to get a granted access from the CrossKnowledge authorization Server.
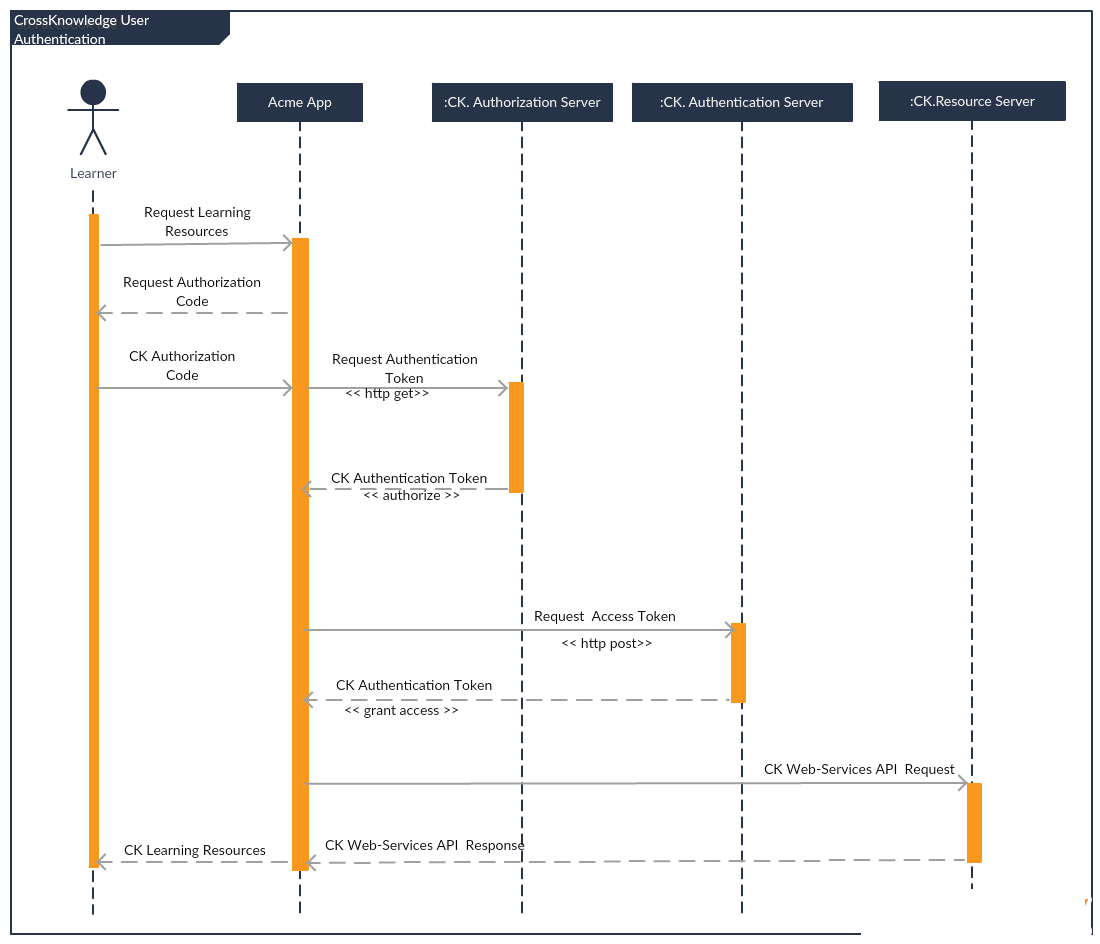
2. Application authorization based on user identifier.
In this scenario, your application must use the user identifier to get an authorization token from the CrossKnowledge Authorization Server.
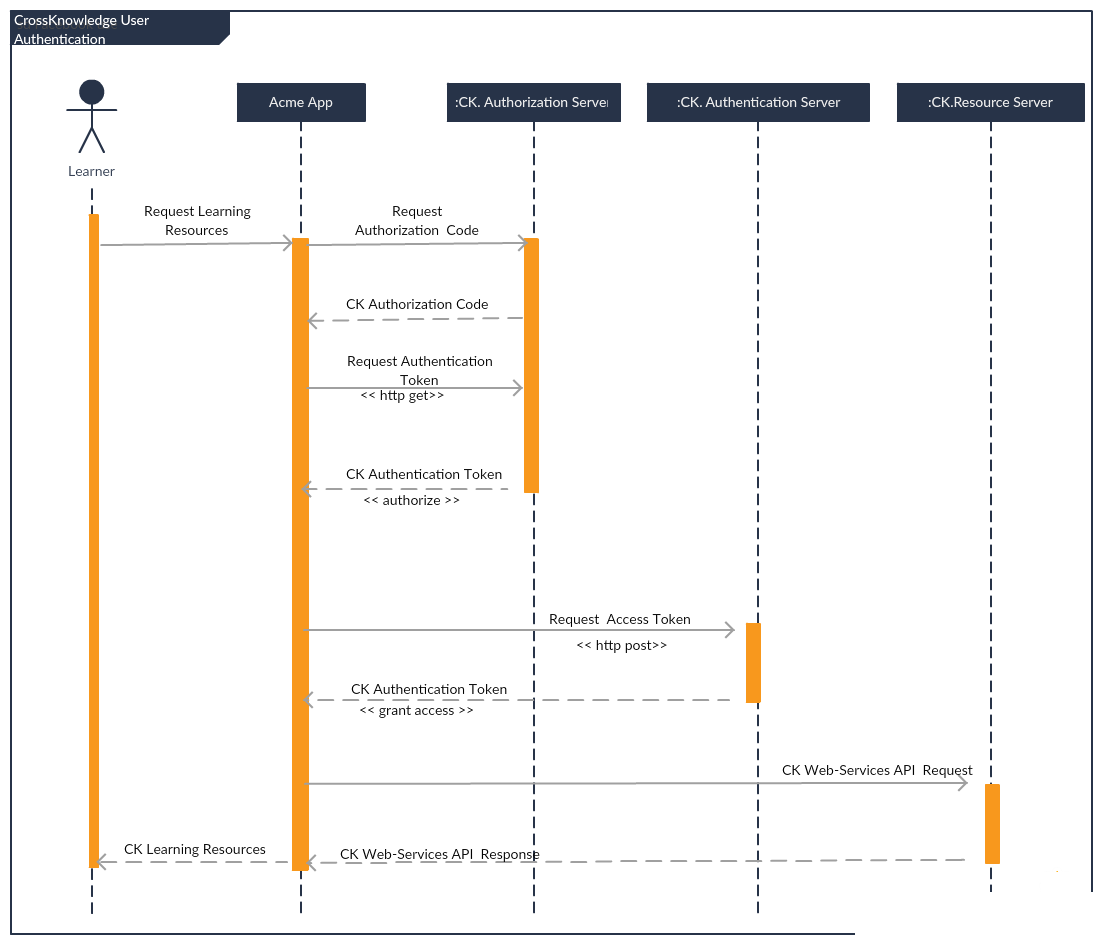
CKAuth web-services.
To obtain an authentication token and an access token to the CrossKnowedge Learner API, your application must use the following CKAuth. web-services:
- Get Authorization Token:
- Get Authentication Token:
- Get Access Token:
Extract access token from authentication server response.
To use the CrossKnowledge Learner API web-services, you'll need to extract the access token from the authentication server then set your access token as a cookie in your HTTP Request Header.
The following code samples show how to extract the access token when the authentication was a success.
Endpoint : API/v1/REST/Learner/mobileLogin.json
Description:
This web-service authenticates the learner by creating a HTTP Session on the server.
$curl = curl_init();
$cookie = "";
curl_setopt($curl, CURLOPT_SSL_VERIFYHOST, 0);
curl_setopt($curl, CURLOPT_SSL_VERIFYPEER, 0);
curl_setopt($curl, CURLOPT_HEADER, 1);
curl_setopt_array($curl, array(
CURLOPT_URL => "https://yourdomain.crossknowledge.com/API/v1/REST/Learner/mobileLogin.json",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => "",
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 30,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "POST",
CURLOPT_POSTFIELDS => "[email protected]&password=bvupehp5z42fwj9uxyxv&deviceid=iphone10",
CURLOPT_HTTPHEADER => array(
"cache-control: no-cache",
"content-type: application/x-www-form-urlencoded",
),
));
$response = curl_exec($curl);
$err = curl_error($curl);
//Grab access token from authentication server HTTP Response.
if ($err) {
echo "cURL Error #:" . $err;
} else {
// get access token
preg_match_all('/^Set-Cookie:\s*([^;]*)/mi', $response, $m);
$cookie = $m[1][3];
}
//Call out a CrossKnowedge Learner API Web-Service by setting the access token in the HTTP Request Header.
curl_setopt($curl, CURLOPT_SSL_VERIFYHOST, 0);
curl_setopt($curl, CURLOPT_SSL_VERIFYPEER, 0);
curl_setopt($curl, CURLOPT_COOKIE, $cookie);
curl_setopt_array($curl, array(
CURLOPT_URL => "https://yourdomain.crossknowledge.com/API/v1/REST/Learner/[email protected]",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => "",
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 30,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "GET",
CURLOPT_HTTPHEADER => array(
"cache-control: no-cache",
),
));
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo "cURL Error #:" . $err;
} else {
echo $response;
}
public void call()
{
var client = new RestClient("https://yourdomain.crossknowledge.com/API/v1/REST/Learner/mobileLogin.json");
var request = new RestRequest(Method.POST);
request.AddHeader("cache-control", "no-cache");
request.AddHeader("content-type", "application/x-www-form-urlencoded");
request.AddParameter("application/x-www-form-urlencoded", "[email protected]&password=bvupehp5z42fwj9uxyxv&deviceid=iphone10", ParameterType.RequestBody);
IRestResponse response = client.Execute(request);
//Extract access token from authentication server HTTP Reponse Header
string header = response.Headers[5].ToString();
string pattern = "(EasyquizzServerSID=[a-z0-9]+)(?!.*EasyquizzServerSID=[a-z0-9])"; // Variable Name 1
var cookieAuthName = "";
var cookieAuthValue = "";
//Get access token
foreach (Match m in Regex.Matches(header, re2))
{
string[] cookieJar = m.Value.Split('=');
cookieAuthName = cookieJar[0];
cookieAuthValue = cookieJar[1];
}
//Call out a CrossKnowedge Learner API Web-Service by setting the access token in the HTTP Request Header.
Uri dn = new Uri("https://yourdomain.crossknowledge.com/API/v1/REST/Learner/[email protected]");
client.BaseUrl = dn;
request.Method = Method.GET;
request.AddHeader("cache-control", "no-cache");
request.AddParameter(cookieAuthName, cookieAuthValue, ParameterType.Cookie);
response = client.Execute(request);
Console.Write(response.Content.ToString());
Console.ReadLine();
}